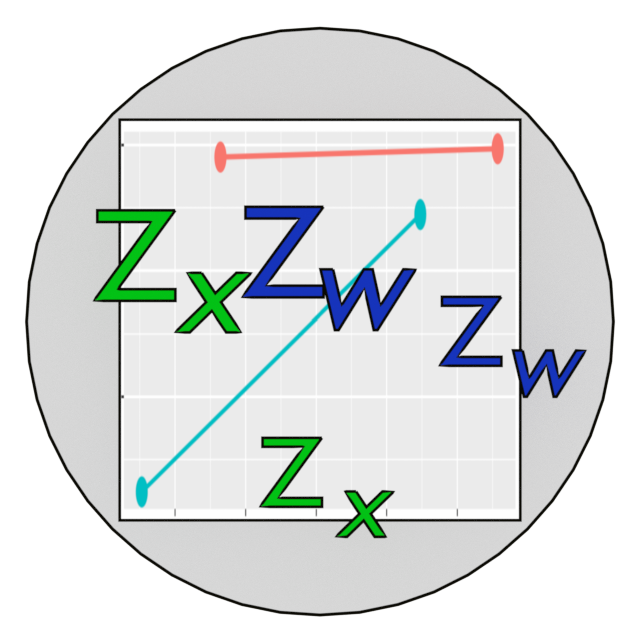
Standardized Moderation Effect and Its Bootstrap CI in 'lavaan'
Source:R/stdmod_lavaan.R
stdmod_lavaan.Rd
Compute the standardized moderation effect in a structural
equation model fitted by lavaan::lavaan()
or its wrappers and
form the nonparametric bootstrap confidence interval.
Usage
stdmod_lavaan(
fit,
x,
y,
w,
x_w,
standardized_x = TRUE,
standardized_y = TRUE,
standardized_w = TRUE,
boot_ci = FALSE,
boot_out = NULL,
R = 100,
conf = 0.95,
use_old_version = FALSE,
...
)
Arguments
- fit
The SEM output by
lavaan::lavaan()
or its wrappers.- x
The name of the focal variable in the model, the variable with its effect on the outcome variable being moderated.
- y
The name of the outcome variable (dependent variable) in the model.
- w
The name of the moderator in the model.
- x_w
The name of the product term (x * w) in the model. It can be the variable generated by the colon operator, e.g.,
"x:w"
, which is only in the model and not in the original data set.- standardized_x
If
TRUE
, the default,x
is standardized when computing the standardized moderation effect.- standardized_y
If
TRUE
, the default,y
is standardized when computing the standardized moderation effect.- standardized_w
If
TRUE
, the default,w
is standardized when computing the standardized moderation effect.- boot_ci
Boolean. Whether nonparametric bootstrapping will be conducted. Default is
FALSE
.- boot_out
If set to the output of
manymome::do_boot()
, the stored bootstrap estimates will be retrieved to form the bootstrap confidence interval. If set, bootstrap estimates stored infit
, if any, will not be used. Default isNULL
.- R
(Not used in the current version. Used when
use_old_version
is set toTRUE
.) The number of nonparametric bootstrapping samples. Default is 100. Set this to at least 2000 in actual use.- conf
The level of confidence. Default is .95, i.e., 95%.
- use_old_version
If set to
TRUE
, it will use the bootstrapping method used in 0.2.7.4 or before. Included only for reproducing previous results if necessary. Default isFALSE
.- ...
(Not used in the current version. Used when
use_old_version
is set toTRUE
.) Optional arguments to be passed toboot::boot()
. Parallel processing can be used by adding the appropriate arguments inboot::boot()
.
Value
A list of class stdmod_lavaan
with these elements:
stdmod
: The standardized moderation effect.ci
: The nonparametric bootstrap confidence interval.NA
if confidence interval not requested.boot_out
: The raw output fromboot::boot()
.NA
if confidence interval not requested.fit
: The original fit object.
Details
Important Notes
Starting from Version 0.2.7.5, of stdmod_lavaan()
adopts an approach
to bootstrapping different from that in the previous
versions (0.2.7.4 and before),
yielding bootstrapping results different from those in
previous versions (for reasons explained later).
To reproduce results from the older version of this function,
set use_old_version
to TRUE
.
How it works
stdmod_lavaan()
accepts a lavaan::lavaan object, the
structural equation model output returned
by lavaan::lavaan()
and its wrappers (e.g, lavaan::sem()
) and computes
the standardized moderation effect using the formula in the appendix of
Cheung, Cheung, Lau, Hui, and Vong (2022).
The standard deviations of the focal variable (the variable with its effect
on the outcome variable being moderated), moderator, and outcome
variable (dependent variable) are computed from the implied
covariance matrix returned by
lavaan::lavInspect()
. Therefore, models fitted to data sets with missing
data (e.g., with missing = "fiml"
) are also supported.
Partial standardization can also be requested. For example, standardization can be requested for only the focal variable and the outcome variable.
There are two ways to request nonparametric bootstrap
confidence interval. First, the model is fitted with se = "boot"
or se = "bootstrap"
in lavaan
. The stored bootstrap
estimates will then be retrieved automatically to compute the
standardized moderation effect. This is the most efficient approach
if the bootstrap confidence intervals are also needed for
other parameters in the model. Bootstrapping needs to be
done only once.
Second, bootstrap estimates can be generated by manymome::do_boot()
.
The output is then supplied through the argument boot_out
.
Bootstrapping also only needs to be done once. This approach
is appropriate when bootstrapping confidence intervals are
not needed for other model parameters, or another type
of confidence interval is needed when fitting the model.
Please refer to the help page of manymome::do_boot()
on how to use this function.
In both approaches, the standard deviations are also computed in each bootstrap samples. This ensures that the sampling variability of the standard deviations is also taken into account in computing the bootstrap confidence interval of the standardized moderation effect.
Note on the differences between the current version (Version 0.2.7.5 or later) and previous versions (0.2.7.4 and before)
In older versions, stdmod_lavaan()
does not allow for
partial standardization. Moreover,
it uses boot::boot()
to do the bootstrapping. Even with
the same seed, the results from boot::boot()
are not
identical to those of lavaan
with se = "boot"
because they differ in the way the indices
of resampling are generated. Both approaches are correct,
They just use the generated random numbers differently.
To have results consistent with those from lavaan
,
the current version of stdmod_lavaan()
adopts a
resampling algorithm identical to that of lavaan
.
Last, in older versions, stdmod_lavaan()
does
bootstrapping every time it is called. This is
inefficient.
The bootstrapping results in the current version are not
identical to those in older versions due to the use
of different resampling algorithms, To reproduce
previous results, set use_old_version
to TRUE
References
Cheung, S. F., Cheung, S.-H., Lau, E. Y. Y., Hui, C. H., & Vong, W. N. (2022) Improving an old way to measure moderation effect in standardized units. Health Psychology, 41(7), 502-505. doi:10.1037/hea0001188
Author
Shu Fai Cheung https://orcid.org/0000-0002-9871-9448
Examples
#Load a test data of 500 cases
dat <- test_mod1
library(lavaan)
mod <-
"
med ~ iv + mod + iv:mod + cov1
dv ~ med + cov2
"
fit <- sem(mod, dat)
# Compute the standardized moderation effect
out_noboot <- stdmod_lavaan(fit = fit,
x = "iv",
y = "med",
w = "mod",
x_w = "iv:mod")
out_noboot
#>
#> Call:
#> stdmod_lavaan(fit = fit, x = "iv", y = "med", w = "mod", x_w = "iv:mod")
#>
#> Variable
#> Focal Variable iv
#> Moderator mod
#> Outcome Variable med
#> Product Term iv:mod
#>
#> lhs op rhs est se z pvalue ci.lower ci.upper
#> Original med ~ iv:mod 0.257 0.025 10.169 0 0.208 0.307
#> Standardized med ~ iv:mod 0.440 NA NA NA NA NA
# Compute the standardized moderation effect and
# its percentile confidence interval using
# nonparametric bootstrapping
# Fit the model with bootstrap confidence intervals
# At least 2000 bootstrap samples should be used
# in real research. 50 is used here only for
# illustration.
fit <- sem(mod, dat, se = "boot", bootstrap = 50,
iseed = 89574)
out_boot <- stdmod_lavaan(fit = fit,
x = "iv",
y = "med",
w = "mod",
x_w = "iv:mod",
boot_ci = TRUE)
out_boot
#>
#> Call:
#> stdmod_lavaan(fit = fit, x = "iv", y = "med", w = "mod", x_w = "iv:mod",
#> boot_ci = TRUE)
#>
#> Variable
#> Focal Variable iv
#> Moderator mod
#> Outcome Variable med
#> Product Term iv:mod
#>
#> lhs op rhs est se z pvalue ci.lower ci.upper
#> Original med ~ iv:mod 0.257 0.033 7.725 0 0.173 0.297
#> Standardized med ~ iv:mod 0.440 NA NA NA 0.316 0.520
#>
#> Confidence interval of standardized moderation effect:
#> - Level of confidence: 95%
#> - Bootstrapping Method: Nonparametric
#> - Type: Percentile
#> - Number of bootstrap samples requests:
#> - Number of bootstrap samples with valid results: 50
#>
#> NOTE: Bootstrapping conducted by the method in 0.2.7.5 or later. To use
#> the method in the older versions for reproducing previous results, set
#> 'use_old_version' to 'TRUE'.